Android Picasso
Introduction
Picasso is a library for managing images on Android and is very simple to use
Picasso.with(getContext()).load(sampleURI).into(imageView)
- Flexible Source Locations
- Caching
- Image Trnasformations
- Error Handling
- Logging
- Request Management
To add it to our projects we simply add it to the gradle. At the time this was
implementation 'com.squareup.picasso:picasso:2.71828'
Resources for this can be found at
https://github.com/alex-wolf-ps/android-picasso
Comparison
Here are some comparisons on the competition at the time, Glide and Fresco. I guess I am more interested in the items to compare as this points to the problem people are trying to solve.
Loading Images
Sources
Picasso supports loading images from either
- String, "https:://www.bibble.co.nz/myimage.png
- Resource ID, images stored in the app drawable e.g. R.drawable.myimage
- URI, Maybe an image from the phone using an intent
- File, a Android File Object
File Types
Picasso supports many file types include JPEG, PNG and SVG. JPEG and PNG are raster images and SVGs are vector Images. Vector images are stored as a series of paths curves and points. Where as raster images of pixels which makes them less unable to scale
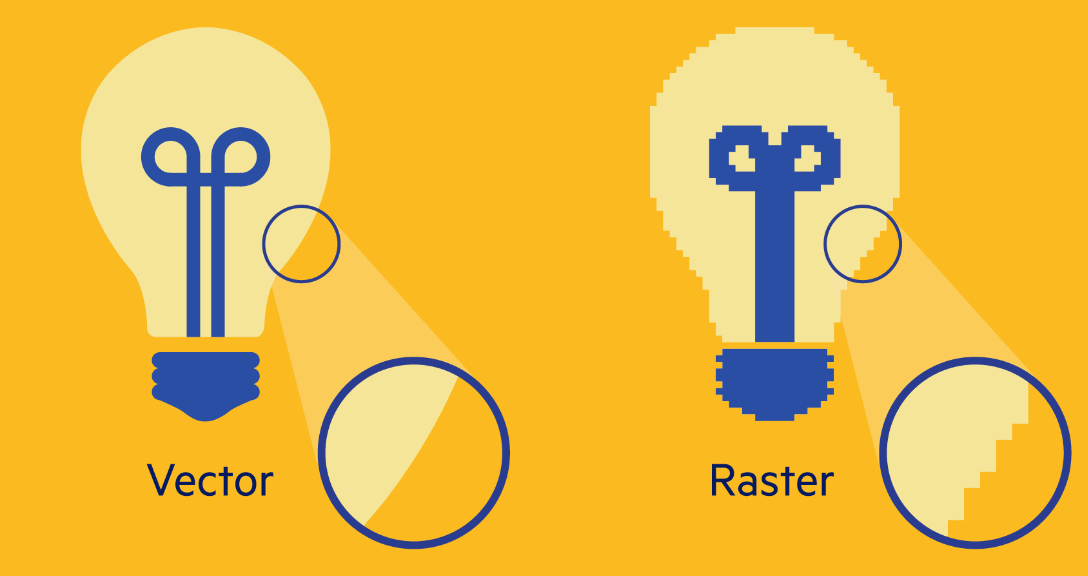
Example 1 Drawable PNG
Create drawables in your res/drawable folder. We can create an appropriate folder for different resolutions e.g.
- /drawable-ldpi For low density screens.
- /drawable-mdpi For medium density screens.
- /drawable-hdpi For high resolution screens.
- /drawable-xhdpi For extra high resolution screens.
Note that the these folders are at the same level as drawable and not inside. From there it is a simple case of getting the Resource ID and loading it into the control.
ImageView banner = view.findViewById(R.id.banner);
Picasso.with(getContext()).load(R.drawable.beans).into(banner);
Example 2 Drawable Icon
Using the code
ImageView imgAboutOne = view.findViewById(R.id.icon_wait);
Picasso.with(getContext()).load(R.drawable.ic_done).into(imgAboutOne);
I struggled to get this to work and got
Failed to create image decoder with message 'unimplemented'
To resolve this I updated to